
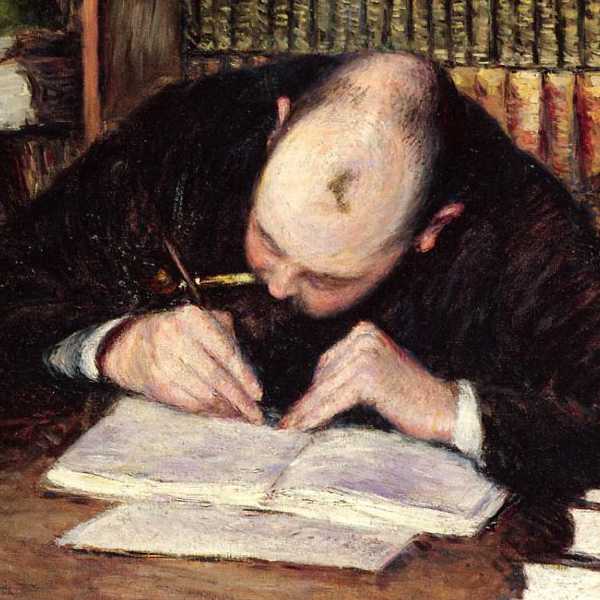
My own gut feeling (and I’m not a particularly astute market-understander) is that unless there is some spectacular failure, then simply how much the owning class wants to believe that AI can bring about a world where capital owners never have to pay for human cognitive or creative labour ever again would keep the money firehose going for very, very long.
(I completely agree, btw, with the observation about how the AI industry has made tech synonymous with “monstrous assholes” in a non-trivial chunk of public consciousness.)
I read that differently.
I parsed it as having the bot only output “sorry, I can’t answer your question”. Ever.